json to graphql typesystem
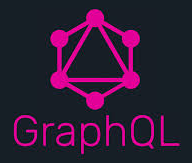
Command Line Interface to convert legacy JSON/REST data to the start of a graphQL schema.
For example, turn this Star Wars SWAPI.co data
{
"name": "Luke Skywalker",
"height": "172",
"mass": "77",
"hair_color": "blond",
"skin_color": "fair",
"eye_color": "blue",
"birth_year": "19BBY",
"gender": "male",
"homeworld": "https://swapi.co/api/planets/1/",
"films": [
"https://swapi.co/api/films/2/",
"https://swapi.co/api/films/6/",
"https://swapi.co/api/films/3/",
"https://swapi.co/api/films/1/",
"https://swapi.co/api/films/7/"
],
"species": [
"https://swapi.co/api/species/1/"
],
"vehicles": [
"https://swapi.co/api/vehicles/14/",
"https://swapi.co/api/vehicles/30/"
],
"starships": [
"https://swapi.co/api/starships/12/",
"https://swapi.co/api/starships/22/"
],
"created": "2014-12-09T13:50:51.644000Z",
"edited": "2014-12-20T21:17:56.891000Z",
"url": "https://swapi.co/api/people/1/"
}
into this:
type people {
name: String
height: String
mass: Date
hair_color: String
skin_color: String
eye_color: String
birth_year: String
gender: String
homeworld: Id
films: [Id]
species: [Id]
vehicles: [Id]
starships: [Id]
created: Date
edited: Date
url: Id
}
It also handles nested data with arrays or objects. e.g. (comments added)
type rootType_belongs_to_collection { // type inside rootType.belongs_to_collection
id: Int
name: String
poster_path: String
backdrop_path: String
}
type rootType_genres { // type inside rootType.genres[]
id: Int
name: String
}
type rootType {
adult: Boolean
backdrop_path: String
belongs_to_collection: rootType_belongs_to_collection // see type above
budget: Int
genres: [rootType_genres] // see type above
}